20 May 2015
Expanding Lists in Bash
A useful but underused feature of the Bash shell is called Brace Expansion.
It takes a few different forms, but basically, anything within the
{ braces }
is expanded, either as a list:
{apples,oranges,bananas}
,
a numerical sequence:
{1..10}
,
or as characters:
{a..z}
.
TL;DR. You can stop reading here and you'll still have got a powerful feature. There is a little bit more to it, though. Nothing difficult, but there are a few details to understand if you're going to get the best out of them.
(The expanding sequences method is covered in the Expanding Sequences tip. Here, we'll look at expanding lists in text).
Expanding Lists
You can supply a hard-coded, comma-separated list to Bash, and it will expand the whole string with every permutation, in order.
$ echo {George,Ringo,John,Paul}" was a Beatle." George was a Beatle. Ringo was a Beatle. John was a Beatle. Paul was a Beatle. $
Notice that the spacing matters. In the above, everything is a single string, so the brace expansion and the " was a Beatle." are treated as a single piece of text to be expanded. Below, the list is expanded, and the text "was a Beatle." is an entirely unrelated piece of text, which is displayed by itself after the expansion.
$ echo {George,Ringo,John,Paul} "was a Beatle." George Ringo John Paul was a Beatle. $
For some reason, the most common usage that anybody finds for this is the creation of directory structures: "mkdir -p /{usr,opt}/myapp/{etc,bin,conf}
", which expands both {usr,opt}
and {etc,bin,conf}
so that the following directories will be created: /usr/myapp/etc, /usr/myapp/bin, /usr/myapp/conf, /opt/myapp/etc, /opt/myapp/bin, /opt/myapp/conf
.
That's useful, but pretty unimaginative; you can expand anything you like. You can expand Shrek and Gremlins to include their sequels, have the Back to the Future and Toy Story trilogies, and the Star Wars whatever-it-may-be-ology, in a simple list.
#!/bin/bash PS3="Select a movie: " select movie in {Shrek,Gremlins}" "{1,2} \ {"Back To The Future","Toy Story"}" "{1,2,3} \ "Star Wars "{1..9} do echo "You chose: \"$movie\"" doneDownload the movie.sh script
The select
will then get the fully-expanded list of:
- Shrek and Gremlins, 1 and 2
- Back to the Future and Toy Story, 1-3
- Star Wars 1-9
An aside: I've abbreviated "Back to the Future" here as "BTTF" so that it should display correctly even on a narrow display; please read "BTTF" as "Back to the Future"
$ ./movie.sh 1) Shrek 1 8) Toy Story 1 15) Star Wars 5 2) Shrek 2 9) Toy Story 2 16) Star Wars 6 3) Gremlins 1 10) Toy Story 3 17) Star Wars 7 4) Gremlins 2 11) Star Wars 1 18) Star Wars 8 5) BTTF 1 12) Star Wars 2 19) Star Wars 9 6) BTTF 2 13) Star Wars 3 7) BTTF 3 14) Star Wars 4 Select a movie: 6 You chose: "Back To The Future 2" Select a movie: 12 You chose: "Star Wars 2" Select a movie:
Another Common Use
Another common use for the expanded list is a bit of a special case: "{,.bak}
". You can use it to create a backup of a file. For example, using cp
's "verbose" option to show what is being done:
$ ls -l /etc/sysctl.conf* -rw-r--r-- 1 root root 2082 May 20 2012 /etc/sysctl.conf $ sudo cp -v /etc/sysctl.conf{,.bak} '/etc/sysctl.conf' -> '/etc/sysctl.conf.bak' $ ls -l /etc/sysctl.conf* -rw-r--r-- 1 root root 2082 May 20 2012 /etc/sysctl.conf -rw-r--r-- 1 root root 2082 May 18 23:55 /etc/sysctl.conf.bak $
The cp
command expands to: "cp /etc/sysctl.conf /etc/sysctl.conf.bak
", causing a backup of the file to be created.
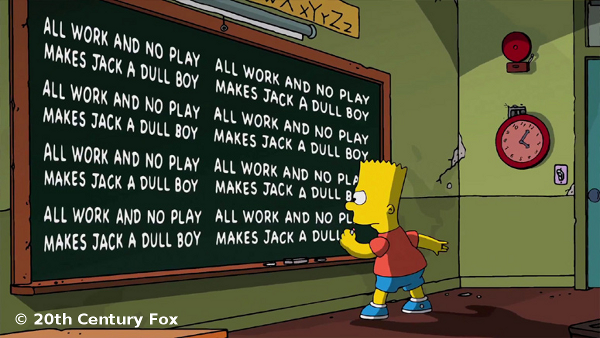
Don't forget to check out the Expanding Sequences method too.
Invest in your career. Buy my Shell Scripting Tutorial today: